Now, it's time to study the next useful widget ever, GtkTable.
GtkTable is a layout widget that contains its children's widgets in a table.
Reference GtkTable
GtkTable has 5 properties:
- Number of columns
- Number of rows
- Column spacing
- Row spacing
- Homogeneous
To create a new table:
GtkWidget *table;
table = gtk_table_new( 2, 2, TRUE );
whereas, the first two params are number of rows and columns in table, the last param indicates whether children should be homogeneous.
The most important part is to handle and pack the children's widgets into the table.
There are two methods we can use to pack:
- gtk_table_attach()
- gtk_table_attach_defaults()
Reference GtkAttachOptions
Now, assume you want to pack a child into the first row, second column, do this:
gtk_table_attach( GTK_TABLE(table), child_widget, 1, 2, 0, 1, GTK_FILL, GTK_FILL, 5, 5 );
The last four params are to specify vertical and horizontal settings including paddings.
Let's do a small practice:
Practice 1: Place 4 label with appropriate coordinate into a table, use defaults attaching settings.
/*
* @author [JaPh]
* @date 23 August, 2009
* @file GtkTable_01.c
* @site http://xjaphx.blogspot.com/
*/
#include <gtk/gtk.h>
int
main ( int argc, char *argv[] )
{
GtkWidget *window;
GtkWidget *table;
GtkWidget *lbl1, *lbl2, *lbl3, *lbl4;
gtk_init( &argc, &argv );
window = gtk_window_new( GTK_WINDOW_TOPLEVEL );
gtk_window_set_title(GTK_WINDOW(window), "[JaPh]Lesson 12: GtkTable");
gtk_window_set_default_size( GTK_WINDOW( window ), 100, 100);
table = gtk_table_new(2, 2, TRUE );
/* set label w/ appropriate coordinates */
lbl1 = gtk_label_new("1,1");
lbl2 = gtk_label_new("1,2");
lbl3 = gtk_label_new("2,1");
lbl4 = gtk_label_new("2,2");
/* attach to table following its coordinates */
gtk_table_attach_defaults( GTK_TABLE(table), lbl1, 0,1,0,1);
gtk_table_attach_defaults( GTK_TABLE(table), lbl2, 1,2,0,1);
gtk_table_attach_defaults( GTK_TABLE(table), lbl3, 0,1,1,2);
gtk_table_attach_defaults( GTK_TABLE(table), lbl4, 1,2,1,2);
gtk_container_add( GTK_CONTAINER(window), table );
g_signal_connect( G_OBJECT(window), "destroy", G_CALLBACK(gtk_main_quit), NULL);
gtk_widget_show_all( window );
gtk_main();
return 0;
}
Practice 2: Let's use this GtkTable layout to do the practice on previous lesson 11.
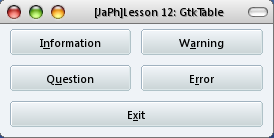
/*
* @author [JaPh]
* @date 23 August, 2009
* @file GtkTable_02.c
* @site http://xjaphx.blogspot.com/
*/
#include <gtk/gtk.h>
/* declare a message box widget */
GtkWidget *msg_box;
/* construct a message box dialog */
void
MessageBox( GtkMessageType mType, GtkButtonsType bType, const gchar *title,
const gchar *msg, const gchar *details )
{
/* message box response code */
int res;
/* create a message box dialog */
msg_box = gtk_message_dialog_new( NULL,
GTK_DIALOG_DESTROY_WITH_PARENT,
mType, bType, msg );
/* set message box title */
gtk_window_set_title( GTK_WINDOW( msg_box ), title );
/* set detail text */
gtk_message_dialog_format_secondary_text( GTK_MESSAGE_DIALOG( msg_box ), details );
/* run the message box dialog */
res = gtk_dialog_run( GTK_DIALOG( msg_box ) );
/* check for dialog response and handle */
if( res == GTK_RESPONSE_OK )
gtk_widget_destroy( msg_box );
}
void
btn_info_clicked( GtkButton *btn, gpointer data )
{
MessageBox( GTK_MESSAGE_INFO, GTK_BUTTONS_OK, "[JaPh] Dialog: INFO" ,
"GtkMessageDialog: Info", "Option: GTK_FILL; Spacing: 5,5; Coord: 0,0" );
}
void
btn_warn_clicked( GtkButton *btn, gpointer data )
{
MessageBox( GTK_MESSAGE_WARNING, GTK_BUTTONS_OK, "[JaPh] Dialog: WARNING",
"GtkMessageDialog: Warning", "Option: GTK_FILL; Spacing: 5,5; Coord: 0,1" );
}
void
btn_ques_clicked( GtkButton *btn, gpointer data )
{
MessageBox( GTK_MESSAGE_QUESTION, GTK_BUTTONS_OK, "[JaPh] Dialog: QUESTION",
"GtkMessageDialog: Question", "Option: GTK_FILL; Spacing: 5,5; Coord: 1,0" );
}
void
btn_erro_clicked( GtkButton *btn, gpointer data )
{
MessageBox( GTK_MESSAGE_ERROR, GTK_BUTTONS_OK, "[JaPh] Dialog: ERROR",
"GtkMessageDialog: Error", "Option: GTK_FILL; Spacing: 5,5; Coord: 1,1" );
}
int
main( int argc, char *argv[] )
{
GtkWidget *window;
GtkWidget *table;
GtkWidget *btn_info, *btn_warn,
*btn_ques, *btn_erro;
GtkWidget *btn_exit;
gtk_init( &argc, &argv );
window = gtk_window_new( GTK_WINDOW_TOPLEVEL );
gtk_window_set_title( GTK_WINDOW(window), "[JaPh]Lesson 12: GtkTable" );
gtk_window_set_default_size( GTK_WINDOW(window), 300, 300 );
table = gtk_table_new( 3, 2, TRUE );
btn_info = gtk_button_new_with_mnemonic("I_nformation");
btn_warn = gtk_button_new_with_mnemonic("W_arning");
btn_ques = gtk_button_new_with_mnemonic("Q_uestion");
btn_erro = gtk_button_new_with_mnemonic("E_rror");
btn_exit = gtk_button_new_with_mnemonic("E_xit");
gtk_table_attach( GTK_TABLE(table), btn_info,
0, 1, 0, 1,
GTK_FILL, GTK_FILL,
5, 5 );
gtk_table_attach( GTK_TABLE(table), btn_warn,
1, 2, 0, 1,
GTK_FILL, GTK_FILL,
5, 5 );
gtk_table_attach( GTK_TABLE(table), btn_ques,
0, 1, 1, 2,
GTK_FILL, GTK_FILL,
5, 5 );
gtk_table_attach( GTK_TABLE(table), btn_erro,
1, 2, 1, 2,
GTK_FILL, GTK_FILL,
5, 5 );
gtk_table_attach( GTK_TABLE(table), btn_exit,
0, 2, 2, 3,
GTK_FILL | GTK_EXPAND, GTK_FILL,
5, 5 );
g_signal_connect( GTK_BUTTON( btn_info ), "clicked",
G_CALLBACK( btn_info_clicked ), NULL );
g_signal_connect( GTK_BUTTON( btn_warn ), "clicked",
G_CALLBACK( btn_warn_clicked ), NULL );
g_signal_connect( GTK_BUTTON( btn_ques ), "clicked",
G_CALLBACK( btn_ques_clicked ), NULL );
g_signal_connect( GTK_BUTTON( btn_erro ), "clicked",
G_CALLBACK( btn_erro_clicked ), NULL );
g_signal_connect( GTK_BUTTON(btn_exit), "clicked",
G_CALLBACK( gtk_main_quit ), NULL );
g_signal_connect( G_OBJECT(window), "destroy",
G_CALLBACK( gtk_main_quit ), NULL );
gtk_container_add( GTK_CONTAINER(window), table );
gtk_widget_show_all( window );
gtk_main();
return 0;
}
It's the end of Lesson 12.
Have fun!@